Build iOS9 Swift-2 Music Video App from scratch using REST-APIs and Step it Up!
Did your last iOS course leave a lot of missing pieces. You understand the basics but still can’t create your own app. Take it to the next level and build Music Video App from scratch using REST-APIs and Step It Up.
Companies expose data over the internet for our applications to consume. Developers have the opportunities to use these APIs within our mobile Apps to solve unique problems. All problems are opportunities in disguise. You can take advantage of the huge amount of data and create powerful mobile applications. Did you know that businesses have been created using REST-APIs like priceline.com, trivago.com, hotels.com, hootsuite.com just to name a few.
This course is going to teach you how to consume REST-APIs, understand JSON and take your knowledge to the next level. We are going to use the iTunes API to get the top 200 Music Videos from the iTunes store. We will build the APP from scratch following the Model-View-Controller design pattern using best coding practices. The following is quick overview of the back-end and user interface processing objectives for our App.
The Music Video App Back-End, User Interface and featured SDK APIs Overview
Back-End Processing
- Handle our network calls on background thread – Grand Central Dispatch.
- Connect to our iTunes REST API and download data – NSURLSession.
- Convert the data to JSON -NSJSONSerialization/JSONObjectWithData
- Parse the data using Swift; no third party APIs.
- Create array of Music Videos using our custom initializer in our class.
- Move the background thread to our Main Queue.
- Pass the array back to our User Interface with completion Handler.
User Interface
- Use the Array of Videos created from our back-end processing as our datasource.
- Use tableview Controller to display our top music videos.
- Create custom cell for our ranking, image and title.
- Create background queue to pull down the image from the URL as we scroll without impacting our user interface.
- Allow the user to scroll up and down and select a specific music video.
- Pass the information selected to another view to display the details.
- Allow the user to play the music video – AVPlayerViewController.
- Allow the user to share through social media – UIActivityViewController.
- Allow the user to go back to the tableview to view more videos – Navigation Controller.
- Give the user the ability to refresh the data using UIRefreshControl.
- Give the user the ability to search on artist or music video title – UISearchController.
- Give the ability to go to a settings page using table view controller using static cells.
- Provide the ability to turn on security using a switch object and saving info to NSUserDefaults.
- If the security switch is on, Touch ID will be required to share info to social media – DeviceOwnerAuthenticationWithBiometrics.
- Allow the user to select the number of music videos to retrieve from our API using a slider object from 10 – 200 and save info to NSUserDefaults.
- Provide the ability to have in-app eMail for customer feedback – MFMailComposeViewController.
Featured SDK
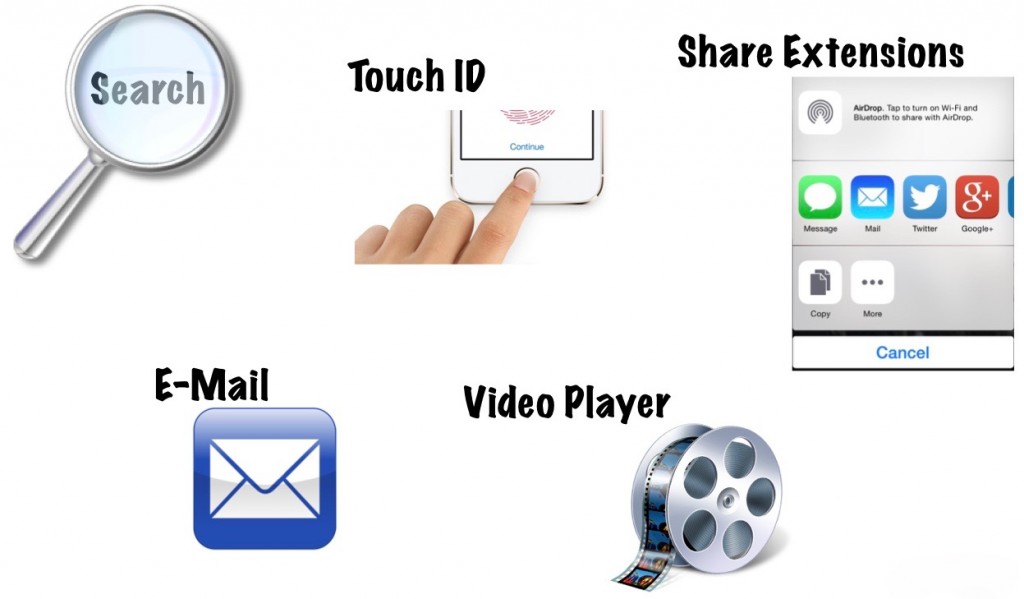
The highlights of what you are going to learn from the iOS SDK Perspective
- Create class for Videos
- Custom initializer in or class
- Create Array object using our Video Class
- TableViewController
- NavigationController
- DataSource/Delegate
- NSUserDefaults
- MFMailComposeViewController
- UISearchResultsUpdating Protocol
- UISearchController
- NSURLSession
- dataTaskWithURL
- reloadData
- toolbar
- Bar Button Items
- UIRefreshControl
- DeviceOwnerAuthenticationWithBiometrics
- NSDictionary
- AVPlayerViewController
- UIAlertController
- NSJSONSerialization
- JSONObjectWithData
- GrandCentralDispatch
- Flexible Space Bar Button
- Image View
- Slider
- Switch
- Custom Cell
- Closures
- NSDATA
- NSNotificationCenter